Table of Contents
Object Oriented Programming, a common phrase today, was originally coined by Alan Kay in the mid 60’s. The concepts of encapsulation were originally pioneered by Kay’s former professor Ivan Sutherland of which his efforts were later improved upon by his successor. Over the next several decades many great minds added innovative techniques that evolved Object Oriented Programming into what we know it as today. These techniques are now considered the fundamental principles of OOP and include: encapsulation, inheritance, abstraction, and polymorphism.
Encapsulation
Encapsulation was the original principle behind Object Oriented Programming. Prior to OOP functional programming shared data openly amongst subroutines. Today object oriented languages encapsulate data and pass objects between one another, these objects are stored in heap memory. Due to heap memory being slower than stack memory these objects are addressed within the heap and referenced within stack memory. This combination is a nice marriage between the two as heap memory has much more space and can dynamically allocate additional blocks of memory as needed, where as stack memory is substantially faster.
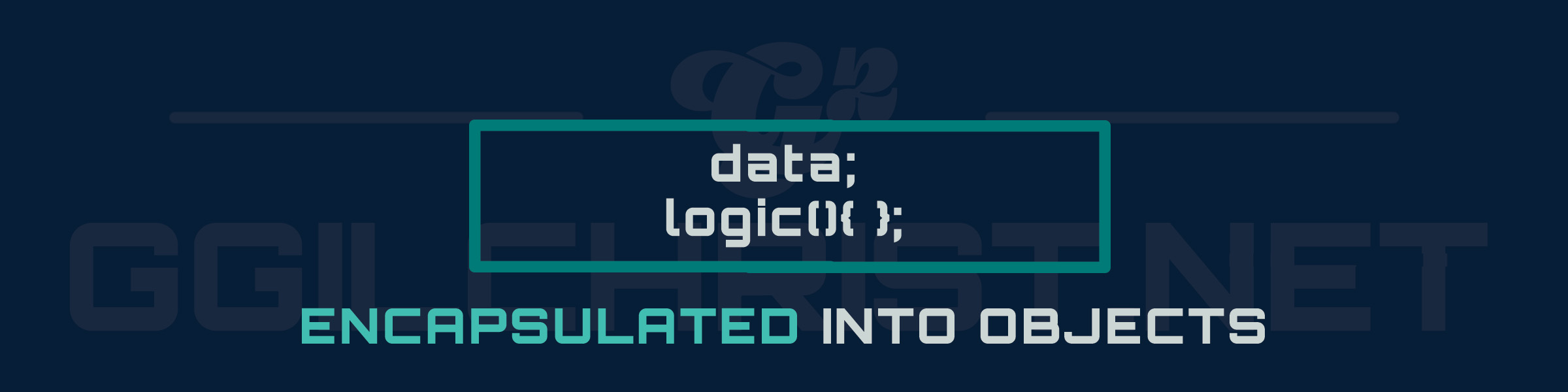
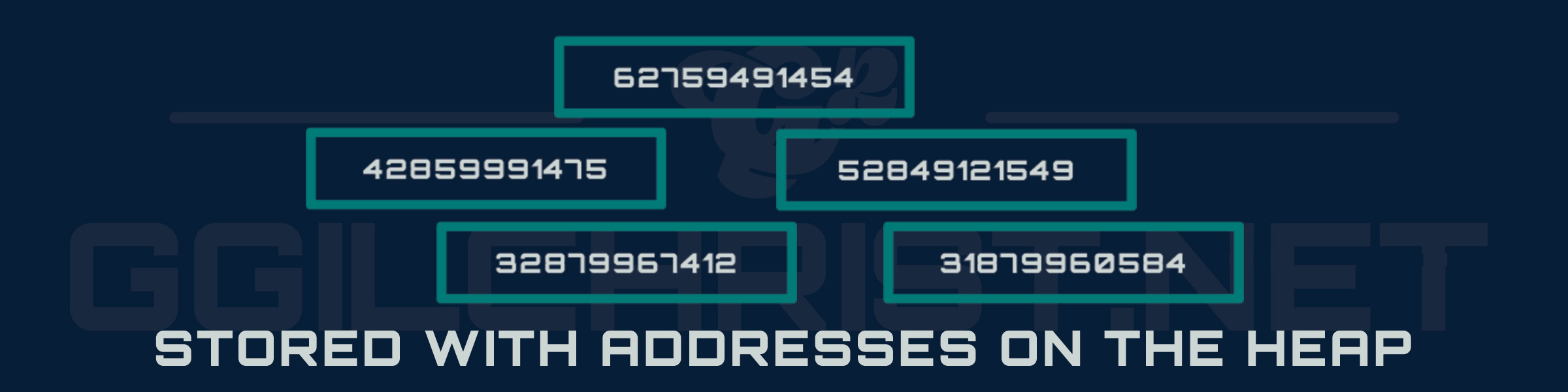
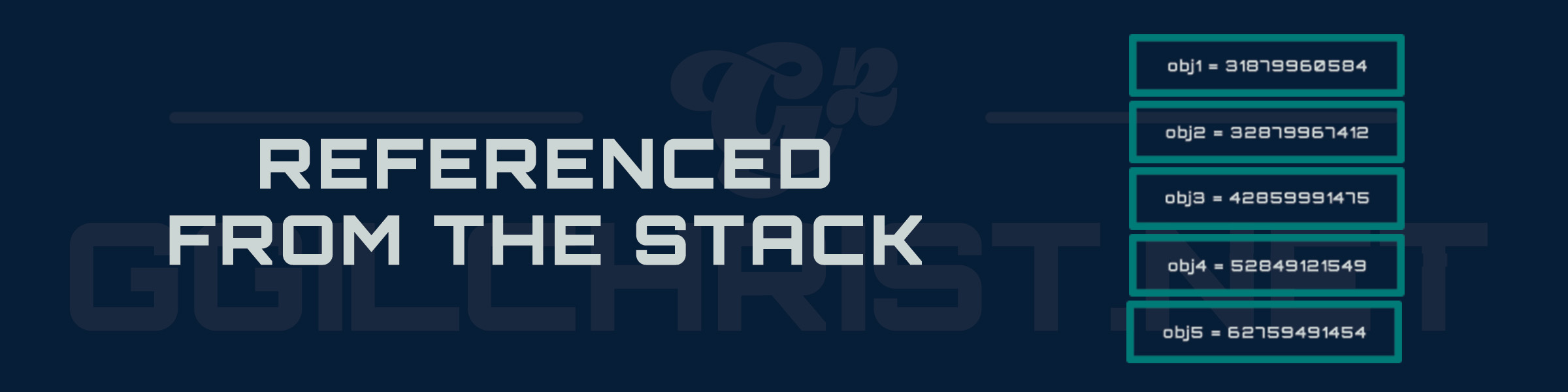
Abstraction
Regarded today as the first Object Oriented Language — Simula — introduced classes and objects as well as access modifiers which limit the access of fields and methods within the object. By hiding the objects members the complexity within the system is reduced. Limiting access to the members encapsulated in the object from other parts of the system and the external world also increases security and stability. Abstraction is a key principle within Object Oriented Programming and should be thoroughly planned when designing the system.
Java offers four unique access modifiers:
- Default – members are only accessible in the same package.
- Public – unrestricted access, members are accessible everywhere
- Protected – members are accessible in the same package or in subclasses of other packages.
- Private – members are only accessible in the same class.
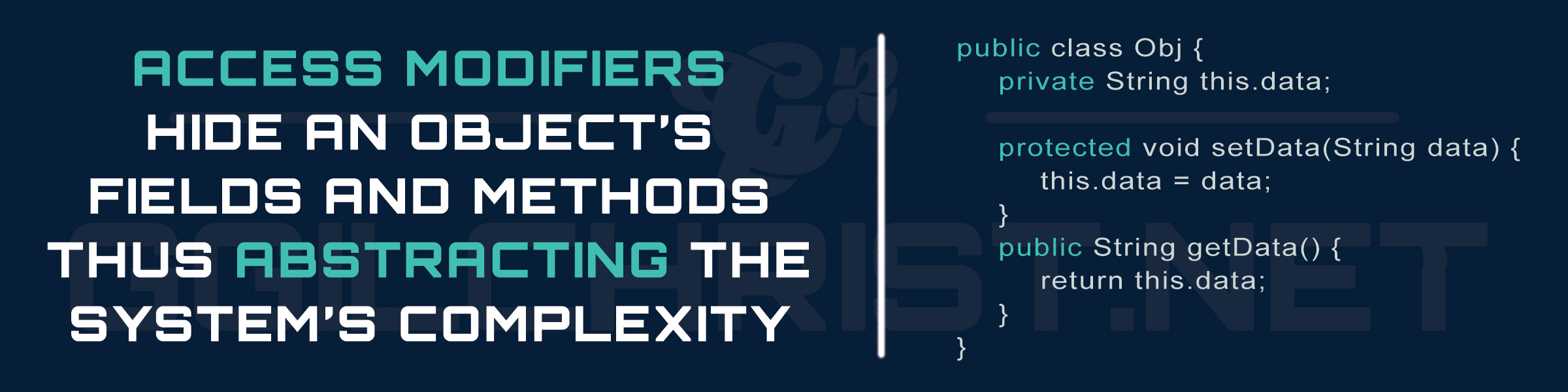
Inheritance
Inheritance was introduced with the creation of Simula 67. Much like how we inherit traits from our parents, a derived class (the child class) inherits fields and methods — known as members — from its base class (the parent class). In OOP we can extend or implement derived classes.
When we extend a derived class we utilize an abstract class, which can not be instantiated, as the base class. The derived class is an extension of the base class. Like an arm that extends from the body, a base class may have many derived classes, but a derived class may only have one base class. These derived classes may inherit the members from the base class.
When we create an interface the derived class must implement the methods that are declared in the base class. It is important to note that methods are declared, not defined in the base class. The purpose of an interface is to create a template for all the derived classes to implement. Like an inherited class an interface’s base class can not be instantiated, however the derived class can implement as many base classes as is needed in an interface.
With the advent of inheritance Object Oriented Languages finally start taking form inevitably becoming the methodology de jure in most software applications.
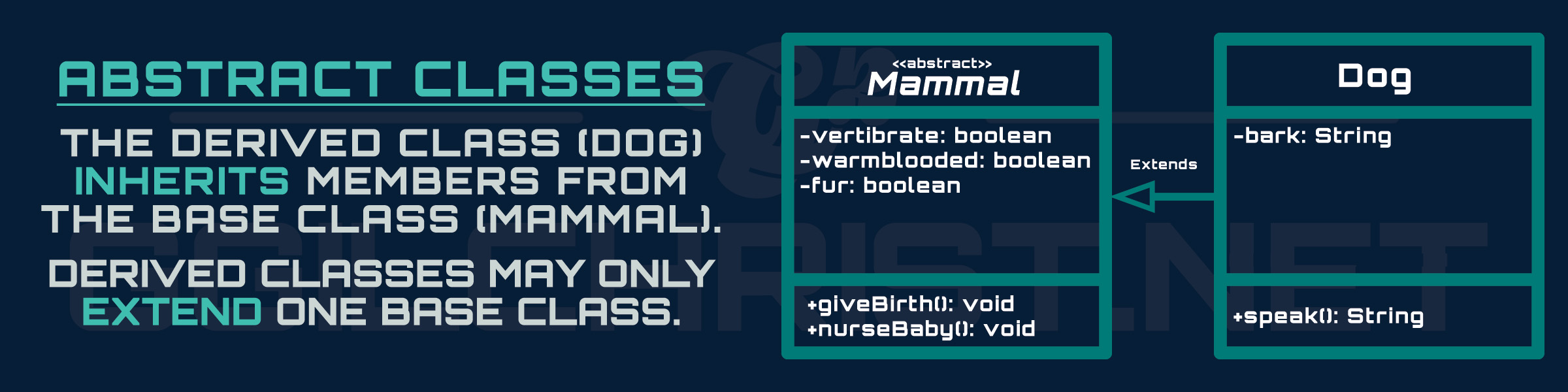
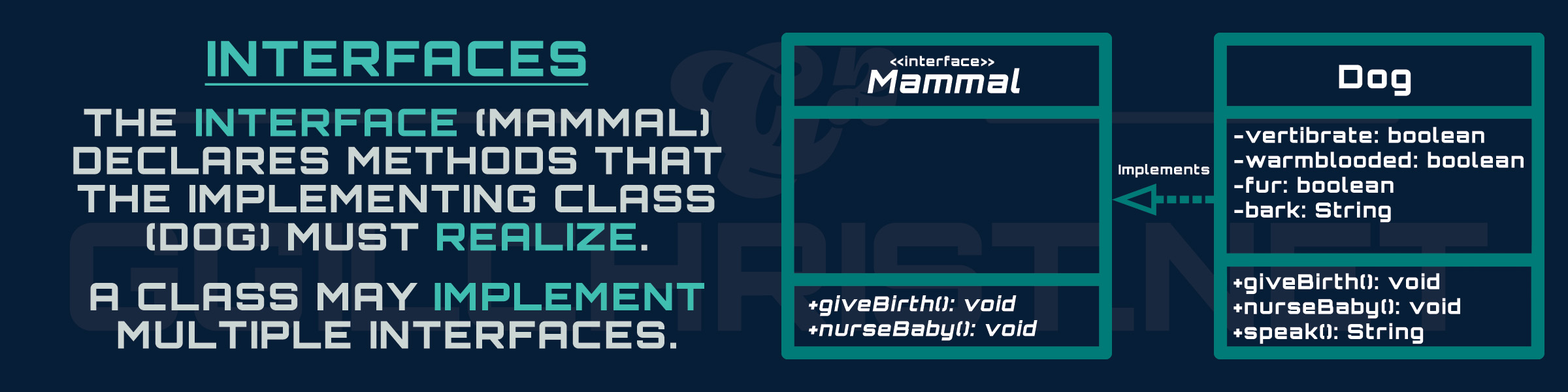
Polymorphism
The Greek word for many forms is polymorphism, it was also a term coined in the mid 80’s by Peter Wegner, crediting Simula for being the first language to implement it. There are two types of polymorphism, overloading and overriding.
When we overload a method we can create alternative functionality of the same method name. We do this in one of three ways: by utilizing different parameter data types (string, integer, boolean, etc.) , by having a different amount of parameters, or by changing the order of the parameters.
The other form of polymorphism is overriding. To override a method we take the default method of an inherited base class and rewrite the method in the derived class, thus changing the functionality. All though it isn’t necessary to use annotation (@Override) when overriding a method in Java it is considered best practice.
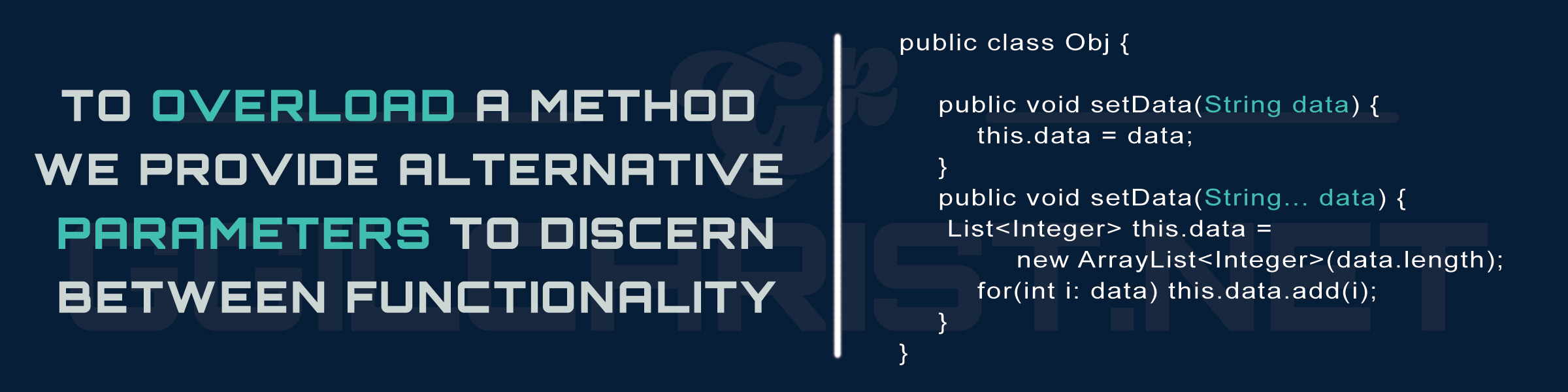
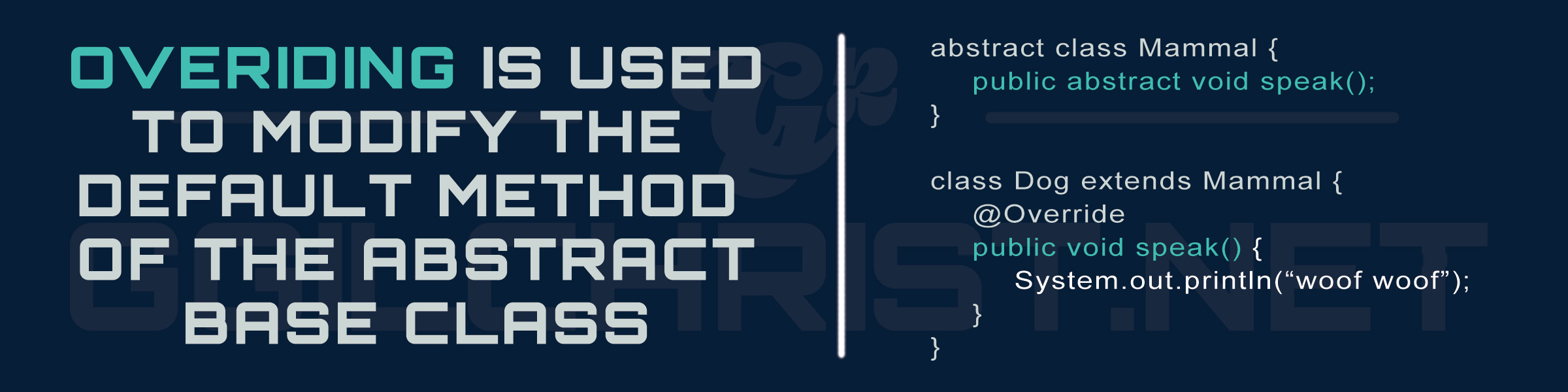
Object Oriented Programming and the great minds that dreamed it have made a lasting impression and will be long remembered in the annals of history within computer science. All though technology has seen many great advances in its short lifetime these principles continue on to this very day. If you are beginning your journey in information technology I hope you learned how valuable these principles will be to you. If you are a seasoned veteran I hope you enjoyed a bit of history on the techniques you so regularly employ. I welcome you to comment below, and hope you enjoy many future blog posts!
2 Responses
Im excited to discover this web site. I want to to thank you for your time just for this fantastic read!! I definitely appreciated every part of it and I have you book marked to look at new information in your site.
I want to to thank you for this very good read!! I certainly enjoyed every little bit of it. I have got you book-marked to check out new stuff you postÖ